Showallocated compute mesh ue5 geometry script
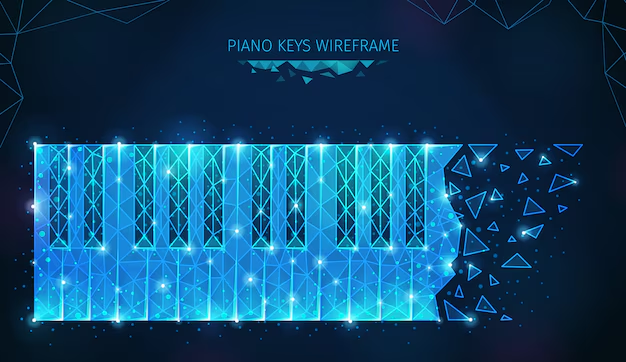
How to Use ShowAllocatedComputeMesh
in Unreal Engine 5 (UE5) Geometry Script
Unreal Engine 5 (UE5) is a powerful game development engine, and one of its standout features is the Geometry Scripting framework, which allows developers to procedurally manipulate and generate 3D assets. The ShowAllocatedComputeMesh
function, often used in conjunction with compute meshes, can help developers debug and optimize geometry scripts by visualizing allocated resources.
In this article, we’ll cover everything you need to know about the ShowAllocatedComputeMesh
function, its role in UE5 Geometry Script, and how to implement it in your projects.
What is a Compute Mesh in UE5?
A compute mesh is a procedural representation of 3D geometry within Unreal Engine. It allows developers to manipulate geometry programmatically using data structures such as:
- Vertex Buffers: Store vertex positions, normals, and tangents.
- Index Buffers: Define the connectivity of vertices (e.g., triangles or polygons).
- Attributes: Such as UVs, vertex colors, and custom data channels.
Compute meshes are commonly used in geometry scripts for generating procedural objects, dynamic meshes, or debugging 3D shapes.
Understanding ShowAllocatedComputeMesh
The ShowAllocatedComputeMesh
function is a debugging tool that displays information about the memory and resources allocated to a compute mesh. When enabled, it helps visualize how much GPU or CPU memory is consumed by a mesh, which is particularly useful for performance optimization.
Key Features:
- Visualizes mesh data such as vertex counts, triangles, and memory usage.
- Helps identify inefficient geometry or overly complex meshes.
- Can be toggled on and off during runtime for debugging purposes.
When Should You Use ShowAllocatedComputeMesh
?
You should use ShowAllocatedComputeMesh
when:
- Debugging Geometry Scripts: Verify if the generated mesh data matches your expectations.
- Optimizing Performance: Identify bottlenecks in the allocation of compute mesh resources.
- Profiling Memory Usage: Understand the memory footprint of complex procedural meshes.
Setting Up a Geometry Script in UE5
To work with ShowAllocatedComputeMesh
, you first need to set up a basic Geometry Script in UE5:
- Enable Geometry Scripting Plugin:
- Go to Edit > Plugins in the UE5 editor.
- Search for “Geometry Scripting” and enable it. Restart the editor if necessary.
- Create a Blueprint or C++ Script:
- In Blueprints, use the Geometry Script node framework.
- In C++, include the necessary Geometry Script headers.
- Set Up a Compute Mesh:
- Use procedural mesh nodes or C++ classes to create and manipulate a compute mesh.
Implementing ShowAllocatedComputeMesh
in a Geometry Script
To implement ShowAllocatedComputeMesh
, follow these steps:
Blueprint Example
- Add a Geometry Script Node:
In your Blueprint, add a Geometry Script Compute Mesh node. - Generate Mesh Data:
Use nodes like Create Grid Mesh, Add Noise to Mesh, or Extrude Polygons to populate the compute mesh. - Enable
ShowAllocatedComputeMesh
:
Add theShowAllocatedComputeMesh
node to your Geometry Script workflow. Connect the output of your compute mesh node to its input. - Preview in the Editor:
Run the script and check the debug visualization in the viewport. You’ll see memory and performance details overlaid on the mesh.
C++ Example
Here’s how you can implement ShowAllocatedComputeMesh
in a C++ Geometry Script:
Practical Use Cases for ShowAllocatedComputeMesh
- Visualizing Complex Meshes: Debugging procedural meshes with thousands of vertices.
- Dynamic Mesh Optimization: Testing performance during runtime updates.
- Debugging Custom Attributes: Ensure UVs, colors, or other data channels are properly allocated.
Tips for Optimizing Compute Mesh Allocations
- Simplify Geometry: Reduce unnecessary vertices and triangles.
- Reuse Buffers: Minimize memory allocations by reusing vertex and index buffers.
- Use LODs: Generate lower-detail versions of meshes for distant objects.
- Profile Regularly: Use tools like
ShowAllocatedComputeMesh
frequently during development.
Troubleshooting Common Issues
- Mesh Not Visible: Ensure the mesh data is properly initialized before calling
ShowAllocatedComputeMesh
. - High Memory Usage: Check for redundant geometry or overly complex procedural operations.
- Script Errors: Verify that all required Geometry Script plugins are enabled.
Conclusion
The ShowAllocatedComputeMesh
function is an invaluable tool for debugging and optimizing compute meshes in UE5. By visualizing resource allocations, you can ensure that your procedural meshes are efficient and performant. Whether you’re a beginner experimenting with geometry scripts or an advanced developer creating complex procedural assets, understanding how to use ShowAllocatedComputeMesh
will elevate your workflows in Unreal Engine 5.